13 February 2025
Introducing cli-utils: A Simple Golang CLI Framework
Reading time: 1m 59s
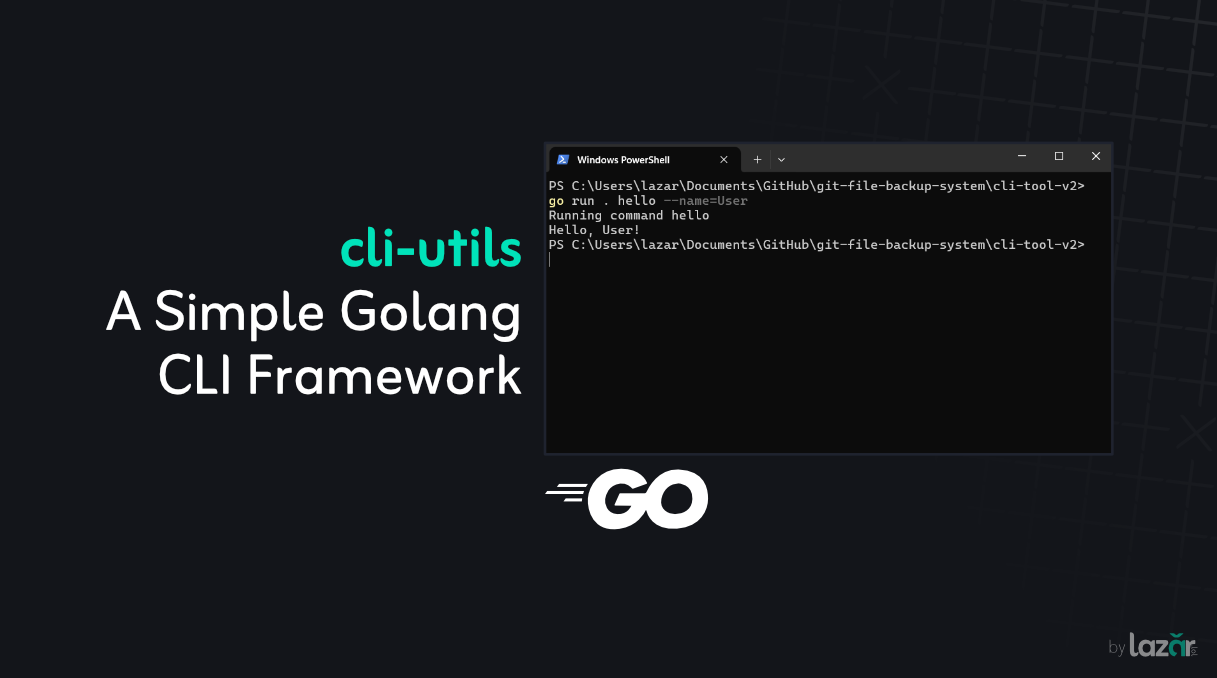
cli-utils is a minimalistic and efficient Go package for building command-line interfaces.
Why cli-utils?
Creating CLI applications in Go often requires boilerplate code for handling commands, arguments, and execution logic. cli-utils simplifies this process by providing a structured way to define and execute commands while ensuring smooth argument validation and error handling.
Installation
Install the package using:
Bash
go get github.com/lazarcloud/cli-utils
How It Works
Here’s an example of how to use cli-utils in a real project:
An example backup CLI tool.
Golang
backup.gopackage main
import (
"cli_backup/cli"
"cli_backup/commands"
)
var COMMANDS = cli.Commands{
commands.HelloCommand,
commands.CheckCommand,
commands.BackupCommand,
commands.DeleteCommand,
commands.DownloadCommand,
}
func main() {
err := cli.NewCLI("cli_backup", COMMANDS, nil).Run()
if err != nil {
panic(err)
}
}
A basic hello commands that says hello to any name.
Golang
commands/hello.gopackage commands
import (
"cli_backup/cli"
"fmt"
)
var HelloCommand = cli.Command{
Name: "hello",
Description: "Prints hello message",
Args: cli.Args{
cli.Arg{
Name: "name",
Description: "Name to say hello to",
Required: false,
Default: "lazar",
},
},
Run: func(args cli.RuntimeArgs, c *cli.CLI) error {
name := args.Get("name")
fmt.Printf("Hello, %s!\n", name)
return nil
},
}
Final words
With cli-utils, you can create structured and maintainable CLI applications with minimal effort. Whether you're building a simple utility or a complex CLI tool, cli-utils provides the foundation you need to streamline development.
Useful Links